In this short article, I will demonstrate how to prevent users from doing Cut, Copy and Paste operations in an ASP.NET TextBox using jQuery. This article is a sample chapter from my EBook called 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls. The chapter content has been modified to publish it as an article. Also please note that for demonstration purposes, I have included JavaScript into the same file. Ideally, these resources should be created in separate folders for maintainability.
The event handling approach in jQuery begins by attaching a handler to an event. The jQuery bind() event method does exactly the same and binds one or more events to a handler. The signature of the bind() method is as follows:
bind(eventType,data,handler)
The parameters of the bind() method are as follows:
eventType is a string holding a JavaScript event type such as click, focus, keyup etc.
data is some optional data you want to pass to the handler
handler is the event handler function which will execute when the event is triggered
Let us quickly jump to the solution and see how we can use the bind() event method to write minimal code to prevent cut, copy and paste operations on the textbox.
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Prevent Cut, Copy and Paste Operations in a TextBoxtitle>
<script type='text/javascript'
src='http://jqueryjs.googlecode.com/files/jquery-1.3.2.min.js'>
script>
<script type="text/javascript">
$(function() {
$('input[id$=tb1]').bind('cut copy paste', function(e) {
e.preventDefault();
alert('You cannot ' + e.type + ' text!');
});
});
script>
head>
<body>
<form id="form1" runat="server">
<div class="bigDiv">
<h2>Prevent Cut, Copy and Paste Operations in a TextBoxh2>
<asp:TextBox ID="tb1" runat="server"
Text="Text which cannot be copied/cut/pasted"
ToolTip="Try Copy/Cut/Paste in textbox"/>
div>
form>
body>
html>
Observe how convenient it is to use jQuery to list multiple events (cut, copy, paste) together and bind it to a handler as shown below.
$('input[id$=tb1]').bind('cut copy paste', function(e) {});
If the user performs any of these events on the textbox (tb1), the default behavior is prevented using e.preventDefault() and the user is alerted with a message. The e.type describes the type of event performed.
$('input[id$=tb1]').bind('cut copy paste', function(e) {
e.preventDefault();
alert('You cannot ' + e.type + ' text!');
});
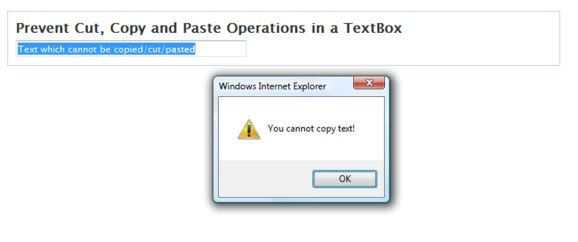
This piece of ‘minimal’ code handles a typical requirement where you are asked to ‘confirm’ an email address and want the user to type it manually, instead of copying and pasting it.
Note: Text can be edited in this textbox, but cut/copy/paste actions are prevented
This demo has been tested on IE7, IE8, Firefox 3, Chrome 2 and Safari 4.
No comments:
Post a Comment