This article demonstrates how to click and retrieve data from a GridView cell. This article is a sample chapter from my EBook called 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls. The chapter has been modified a little to publish it as an article.
Note that for demonstration purposes, I have included jQuery and CSS code in the same page. Ideally, these resources should be created in separate folders for maintainability.
Let us quickly jump to the solution and see how to click and retrieve data from a GridView cell
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Click and retrieve Cell Valuetitle>
<style type="text/css">
.highlite
{
background-color:Gray;
}
style>
<script type="text/javascript"
src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.min.js">
script>
<script type="text/javascript">
$(function() {
$(".gv > tbody > tr:not(:has(table, th))")
.css("cursor", "pointer")
.click(function(e) {
$(".gv td").removeClass("highlite");
var $cell = $(e.target).closest("td");
$cell.addClass('highlite');
var $currentCellText = $cell.text();
var $leftCellText = $cell.prev().text();
var $rightCellText = $cell.next().text();
var $colIndex = $cell.parent().children().index($cell);
var $colName = $cell.closest("table")
.find('th:eq(' + $colIndex + ')').text();
$("#para").empty()
.append("Current Cell Text: "
+ $currentCellText + "
")
")
.append("Text to Left of Clicked Cell: "
+ $leftCellText + "
")
")
.append("Text to Right of Clicked Cell: "
+ $rightCellText + "
")
")
.append("Column Name of Clicked Cell: "
+ $colName)
});
});
script>
head>
<body>
<form id="form1" runat="server">
<div class="tableDiv">
<h2>Click on any of the table cells to retrieve information
about the cell and its surrounding elementsh2><br />
<asp:GridView ID="grdEmployees" runat="server"
AllowPaging="True" PageSize="5" AutoGenerateColumns="False"
DataSourceID="ObjectDataSource1" class="gv">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" />
<asp:BoundField DataField="FName" HeaderText="FName" />
<asp:BoundField DataField="MName" HeaderText="MName" />
<asp:BoundField DataField="LName" HeaderText="LName" />
<asp:BoundField DataField="DOB" HeaderText="DOB"
DataFormatString="{0:MM/dd/yyyy}"/>
<asp:BoundField DataField="Sex" HeaderText="Sex" />
Columns>
asp:GridView>
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetEmployeeList" TypeName="Employee">
asp:ObjectDataSource>
<br />
<p id="para">p>
div>
form>
body>
html>
The ObjectDataSource uses the Employee.cs or Employee.vb class whose definition is as follows:
C#
using System;
using System.Collections.Generic;
public class Employee
{
public int ID { get; set; }
public string FName { get; set; }
public string MName { get; set; }
public string LName { get; set; }
public DateTime DOB { get; set; }
public char Sex { get; set; }
public List<Employee> GetEmployeeList()
{
List<Employee> empList = new List<Employee>();
empList.Add(new Employee() { ID = 1, FName = "John", MName = "", LName = "Shields", DOB = DateTime.Parse("12/11/1971"), Sex = 'M' });
empList.Add(new Employee() { ID = 2, FName = "Mary", MName = "Matthew", LName = "Jacobs", DOB = DateTime.Parse("01/17/1961"), Sex = 'F' });
empList.Add(new Employee() { ID = 3, FName = "Amber", MName = "Carl", LName = "Agar", DOB = DateTime.Parse("12/23/1971"), Sex = 'M' });
empList.Add(new Employee() { ID = 4, FName = "Kathy", MName = "", LName = "Berry", DOB = DateTime.Parse("11/15/1976"), Sex = 'F' });
empList.Add(new Employee() { ID = 5, FName = "Lena", MName = "Ashco", LName = "Bilton", DOB = DateTime.Parse("05/11/1978"), Sex = 'F' });
empList.Add(new Employee() { ID = 6, FName = "Susanne", MName = "", LName = "Buck", DOB = DateTime.Parse("03/7/1965"), Sex = 'F' });
empList.Add(new Employee() { ID = 7, FName = "Jim", MName = "", LName = "Brown", DOB = DateTime.Parse("09/11/1972"), Sex = 'M' });
empList.Add(new Employee() { ID = 8, FName = "Jane", MName = "G", LName = "Hooks", DOB = DateTime.Parse("12/11/1972"), Sex = 'F' });
empList.Add(new Employee() { ID = 9, FName = "Robert", MName = "", LName = "", DOB = DateTime.Parse("06/28/1964"), Sex = 'M' });
empList.Add(new Employee() { ID = 10, FName = "Krishna", MName = "Murali", LName = "Sunkam", DOB = DateTime.Parse("04/18/1969"), Sex = 'M' });
empList.Add(new Employee() { ID = 11, FName = "Cindy", MName = "Preston", LName = "Fox", DOB = DateTime.Parse("06/15/1978"), Sex = 'M' });
empList.Add(new Employee() { ID = 12, FName = "Nicole", MName = "G", LName = "Holiday", DOB = DateTime.Parse("08/21/1974"), Sex = 'F' });
empList.Add(new Employee() { ID = 13, FName = "Sandra", MName = "T", LName = "Feng", DOB = DateTime.Parse("04/15/1976"), Sex = 'F' });
empList.Add(new Employee() { ID = 14, FName = "Roberto", MName = "", LName = "Tamburello", DOB = DateTime.Parse("01/06/1982"), Sex = 'M' });
empList.Add(new Employee() { ID = 15, FName = "Cynthia", MName = "O", LName = "Lugo", DOB = DateTime.Parse("01/21/1968"), Sex = 'M' });
empList.Add(new Employee() { ID = 16, FName = "Yuhong", MName = "R", LName = "Li", DOB = DateTime.Parse("08/22/1979"), Sex = 'M' });
empList.Add(new Employee() { ID = 17, FName = "Alex", MName = "", LName = "Shoop", DOB = DateTime.Parse("03/01/1972"), Sex = 'M' });
empList.Add(new Employee() { ID = 18, FName = "Jack", MName = "K", LName = "Brown", DOB = DateTime.Parse("04/11/1978"), Sex = 'M' });
empList.Add(new Employee() { ID = 19, FName = "Andrew", MName = "U", LName = "Gibson", DOB = DateTime.Parse("08/21/1977"), Sex = 'M' });
empList.Add(new Employee() { ID = 20, FName = "George", MName = "K", LName = "Wood", DOB = DateTime.Parse("07/15/1972"), Sex = 'M' });
empList.Add(new Employee() { ID = 21, FName = "Eugene", MName = "", LName = "Miller", DOB = DateTime.Parse("09/13/1974"), Sex = 'M' });
empList.Add(new Employee() { ID = 22, FName = "Russell", MName = "", LName = "Gorgi", DOB = DateTime.Parse("08/19/1978"), Sex = 'M' });
empList.Add(new Employee() { ID = 23, FName = "Katie", MName = "", LName = "Sylar", DOB = DateTime.Parse("08/21/1978"), Sex = 'M' });
empList.Add(new Employee() { ID = 24, FName = "Michael", MName = "M", LName = "Bentler", DOB = DateTime.Parse("11/26/1977"), Sex = 'M' });
empList.Add(new Employee() { ID = 25, FName = "Barbara", MName = "L", LName = "Duffy", DOB = DateTime.Parse("05/29/1972"), Sex = 'M' });
empList.Add(new Employee() { ID = 26, FName = "Stefen", MName = "J", LName = "Northup", DOB = DateTime.Parse("01/26/1972"), Sex = 'M' });
empList.Add(new Employee() { ID = 27, FName = "Shane", MName = "", LName = "Nay", DOB = DateTime.Parse("02/21/1978"), Sex = 'M' });
empList.Add(new Employee() { ID = 28, FName = "Brenda", MName = "", LName = "Lugo", DOB = DateTime.Parse("08/18/1981"), Sex = 'F' });
empList.Add(new Employee() { ID = 29, FName = "Shammi", MName = "I", LName = "Rai", DOB = DateTime.Parse("03/13/1968"), Sex = 'M' });
empList.Add(new Employee() { ID = 30, FName = "Rajesh", MName = "H", LName = "Vyas", DOB = DateTime.Parse("04/19/1969"), Sex = 'M' });
empList.Add(new Employee() { ID = 31, FName = "Gabe", MName = "P", LName = "Lloyd", DOB = DateTime.Parse("08/21/1971"), Sex = 'M' });
return empList;
}
}
VB.NET
Imports System
Imports System.Collections.Generic
Public Class Employee
Private privateID As Integer
Public Property ID() As Integer
Get
Return privateID
End Get
Set(ByVal value As Integer)
privateID = value
End Set
End Property
Private privateFName As String
Public Property FName() As String
Get
Return privateFName
End Get
Set(ByVal value As String)
privateFName = value
End Set
End Property
Private privateMName As String
Public Property MName() As String
Get
Return privateMName
End Get
Set(ByVal value As String)
privateMName = value
End Set
End Property
Private privateLName As String
Public Property LName() As String
Get
Return privateLName
End Get
Set(ByVal value As String)
privateLName = value
End Set
End Property
Private privateDOB As DateTime
Public Property DOB() As DateTime
Get
Return privateDOB
End Get
Set(ByVal value As DateTime)
privateDOB = value
End Set
End Property
Private privateSex As Char
Public Property Sex() As Char
Get
Return privateSex
End Get
Set(ByVal value As Char)
privateSex = value
End Set
End Property
Public Function GetEmployeeList() As List(Of Employee)
Dim empList As New List(Of Employee)()
empList.Add(New Employee() With {.ID = 1, .FName = "John", .MName = "", .LName = "Shields", .DOB = DateTime.Parse("12/11/1971"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 2, .FName = "Mary", .MName = "Matthew", .LName = "Jacobs", .DOB = DateTime.Parse("01/17/1961"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 3, .FName = "Amber", .MName = "Carl", .LName = "Agar", .DOB = DateTime.Parse("12/23/1971"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 4, .FName = "Kathy", .MName = "", .LName = "Berry", .DOB = DateTime.Parse("11/15/1976"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 5, .FName = "Lena", .MName = "Ashco", .LName = "Bilton", .DOB = DateTime.Parse("05/11/1978"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 6, .FName = "Susanne", .MName = "", .LName = "Buck", .DOB = DateTime.Parse("03/7/1965"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 7, .FName = "Jim", .MName = "", .LName = "Brown", .DOB = DateTime.Parse("09/11/1972"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 8, .FName = "Jane", .MName = "G", .LName = "Hooks", .DOB = DateTime.Parse("12/11/1972"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 9, .FName = "Robert", .MName = "", .LName = "", .DOB = DateTime.Parse("06/28/1964"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 10, .FName = "Krishna", .MName = "Murali", .LName = "Sunkam", .DOB = DateTime.Parse("04/18/1969"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 11, .FName = "Cindy", .MName = "Preston", .LName = "Fox", .DOB = DateTime.Parse("06/15/1978"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 12, .FName = "Nicole", .MName = "G", .LName = "Holiday", .DOB = DateTime.Parse("08/21/1974"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 13, .FName = "Sandra", .MName = "T", .LName = "Feng", .DOB = DateTime.Parse("04/15/1976"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 14, .FName = "Roberto", .MName = "", .LName = "Tamburello", .DOB = DateTime.Parse("01/06/1982"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 15, .FName = "Cynthia", .MName = "O", .LName = "Lugo", .DOB = DateTime.Parse("01/21/1968"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 16, .FName = "Yuhong", .MName = "R", .LName = "Li", .DOB = DateTime.Parse("08/22/1979"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 17, .FName = "Alex", .MName = "", .LName = "Shoop", .DOB = DateTime.Parse("03/01/1972"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 18, .FName = "Jack", .MName = "K", .LName = "Brown", .DOB = DateTime.Parse("04/11/1978"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 19, .FName = "Andrew", .MName = "U", .LName = "Gibson", .DOB = DateTime.Parse("08/21/1977"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 20, .FName = "George", .MName = "K", .LName = "Wood", .DOB = DateTime.Parse("07/15/1972"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 21, .FName = "Eugene", .MName = "", .LName = "Miller", .DOB = DateTime.Parse("09/13/1974"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 22, .FName = "Russell", .MName = "", .LName = "Gorgi", .DOB = DateTime.Parse("08/19/1978"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 23, .FName = "Katie", .MName = "", .LName = "Sylar", .DOB = DateTime.Parse("08/21/1978"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 24, .FName = "Michael", .MName = "M", .LName = "Bentler", .DOB = DateTime.Parse("11/26/1977"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 25, .FName = "Barbara", .MName = "L", .LName = "Duffy", .DOB = DateTime.Parse("05/29/1972"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 26, .FName = "Stefen", .MName = "J", .LName = "Northup", .DOB = DateTime.Parse("01/26/1972"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 27, .FName = "Shane", .MName = "", .LName = "Nay", .DOB = DateTime.Parse("02/21/1978"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 28, .FName = "Brenda", .MName = "", .LName = "Lugo", .DOB = DateTime.Parse("08/18/1981"), .Sex = "F"c})
empList.Add(New Employee() With {.ID = 29, .FName = "Shammi", .MName = "I", .LName = "Rai", .DOB = DateTime.Parse("03/13/1968"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 30, .FName = "Rajesh", .MName = "H", .LName = "Vyas", .DOB = DateTime.Parse("04/19/1969"), .Sex = "M"c})
empList.Add(New Employee() With {.ID = 31, .FName = "Gabe", .MName = "P", .LName = "Lloyd", .DOB = DateTime.Parse("08/21/1971"), .Sex = "M"c})
Return empList
End Function
End Class
The example starts with a filter applied on the GridView rows
$(".gv > tbody > tr:not(:has(table, th))")
This filter is required since a GridView does not render a THEAD and a TFOOT (accessibility tags) by default. For the header, the GridView generates TH’s inside a TR. Similarly for the footer, the GridView generates a TABLE inside a TR and so on. Hence it is required to use additional filters to exclude header and footer rows while performing operations on the GridView rows. The filter shown above selects only those rows which are inside the TBODY.
This recipe uses event delegation. Observe how we have used ‘e.target’ to find out the element that was clicked. This object is cached in the ‘cell’ variable.
var $cell = $(e.target).closest("td");
The closest() as given in the jQuery documentation, “works by first looking at the current element to see if it matches the specified expression, if so it just returns the element itself. If it doesn't match then it will continue to traverse up the document, parent by parent, until an element is found that matches the specified expression.”
With the help of the ‘cell’ variable, we can use the DOM tree traversal methods like prev() and next(), to retrieve the value of the immediate ‘preceding’ and ‘following’ elements, respectively.
var $leftCellText = $cell.prev().text();
var $rightCellText = $cell.next().text();
Similarly the column header text is retrieved using the code shown below.
var $colIndex = $cell.parent().children().index($cell);
var $colName = $cell.closest("table")
.find('th:eq(' + $colIndex + ')').text();
As shown above, after retrieving the column index, we use the closest() method to traverse up the DOM, parent by parent until we find the table element. The Header text is then selected using
('th:eq(' + $colIndex + ')').text()
When a Cell of the GridView is clicked (for example 2nd Row and 3rd Column), the result is as shown below:
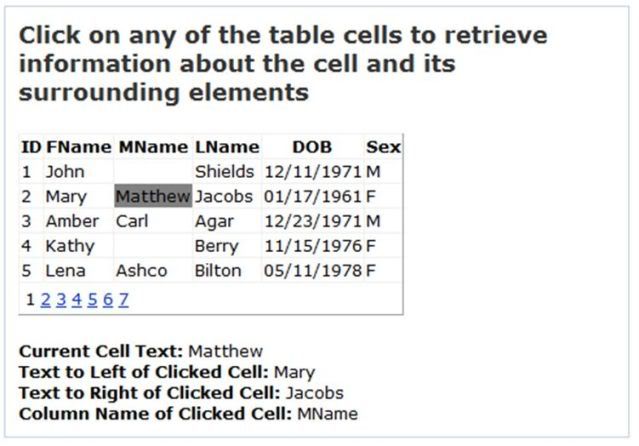
See a Live Demo
I hope you found this article useful and I thank you for viewing it. This article was taken from my EBook called 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls which contains similar recipes that you can use in your applications. The entire source code of this article can be downloaded over here
No comments:
Post a Comment