This article shows you how to limit characters entered in an ASP.NET Multiline TextBox. The ASP.NET Multiline TextBox ignores the MaxLength property. So one of the ways of solving this requirement is to use Client Script and detect if the maximum character limit of the Multiline TextBox has been reached. I will be showing a common technique adopted by developers to solve this requirement. I will then explain why this technique is not user friendly and how to improve on it.
This article is a sample chapter from my EBook called 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls. The chapter content has been modified to publish it as an article. Also please note that for demonstration purposes, I have included both the JavaScript and CSS into the same file. Ideally, these resources should be created in seperate folders for maintainability.
A common technique adopted by developers is to capture the textbox keyup event and calculate the number of characters in the textbox, as the user types in it. If the character exceeds the limit of the textbox (in our case 50 characters), the additional characters entered by the user is disallowed. The code is as shown below:
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Limit Characters in a Multiline TextBoxtitle>
<script type='text/javascript'
src='http://jqueryjs.googlecode.com/files/jquery-1.3.2.min.js'>
script>
<script type="text/javascript">
$(function() {
var limit = 50;
$('textarea[id$=tb1]').keyup(function() {
var len = $(this).val().length;
if (len > limit) {
this.value = this.value.substring(0, limit);
}
$('#spn).text(limit - len + " characters left");
});
});
script>
head>
<body>
<form id="form1" runat="server">
<div>
<asp:TextBox ID="tb1" runat="server" TextMode="MultiLine"/><br />
<span id="spn ">span>
div>
form>
body>
html>
Observe how we are selecting the control using textarea in the code shown above $('textarea[id$=tb1]'). This is as the ASP.NET Multiline TextBox renders as a textarea.
Although the solution given above restricts the user from entering more than 50 characters, this behavior can be confusing for users who may not realize that they have reached the TextBox limit. Instead of disallowing extra characters, a slick way of handling this requirement would be to visually indicate to the user when the textbox limit has been exceeded. Then before submitting the form, give the user a chance to remove the extra text. The code shown below changes the background color of the textbox to red when the textbox limit is exceeded. The user is also prevented from submitting the form.
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Limit Characters in a Multiline TextBoxtitle>
<script type='text/javascript'
src='http://jqueryjs.googlecode.com/files/jquery-1.3.2.min.js'>
script>
<style type="text/css">
.exceeded{
background-color:red;
}
style>
<script type="text/javascript">
$(function() {
var limit = 50;
var tb = $('textarea[id$=tb1]');
$(tb).keyup(function() {
var len = $(this).val().length;
if (len > limit) {
//this.value = this.value.substring(0, 50);
$(this).addClass('exceeded');
$('#spn').text(len - limit + " characters exceeded");
}
else {
$(this).removeClass('exceeded');
$('#spn').text(limit - len + " characters left");
}
});
$('input[id$=btnSubmit]').click(function(e) {
var len = $(tb).val().length;
if (len > limit) {
e.preventDefault();
}
});
});
script>
head>
<body>
<form id="form1" runat="server">
<div class="smallDiv">
<h2>Type into this textbox which accepts 50 characters overallh2>
<asp:TextBox ID="tb1" runat="server" TextMode="MultiLine"/><br />
(This textbox accepts only 50 characters) <br />
<span id="spn">span> <br />
<asp:Button ID="btnSubmit" runat="server" Text="Submit"/>
<span id="error">span>
<br /><br />
Tip: Clicking on the Submit button when number of characters are
less than 50, results in a postback to same page. If you exceed 50
characters, the exceeded characters are printed below the textbox
and a postback is prevented.
div>
form>
body>
html>
When the number of characters exceeds the textbox limit, we add the exceeded class to the textbox, which turns the textbox background to red, indicating the user that the limit has been exceeded. The result is shown here:
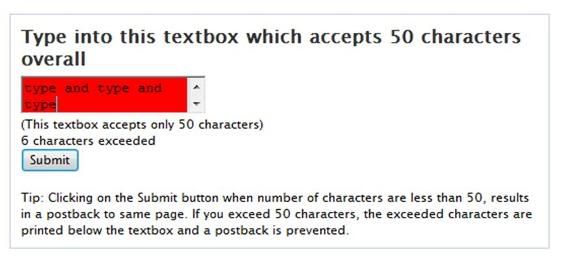
When the user tries and submits the form now, the length of the TextBox is calculated and the user is prevented from submitting the form, since the textbox exceeds the permissible length.
When the user removes the extra text, the exceeded class is removed. The result is shown here:
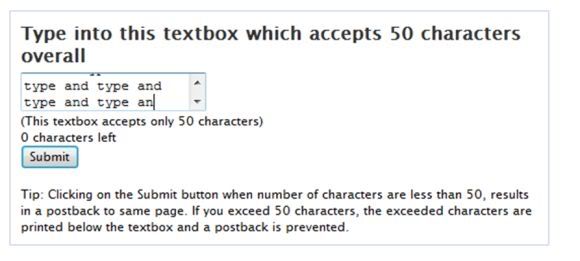
The form can now be submitted. You can see a Live Demo here . This demo has been tested on IE7, IE8, Firefox 3, Chrome 2 and Safari 4.
There are plenty of such similar tips that I will be covering in my upcoming EBook over here 51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls
I hope you liked this article and I thank you for viewing it.
No comments:
Post a Comment